5 things you didn’t know the Javascript Console can do
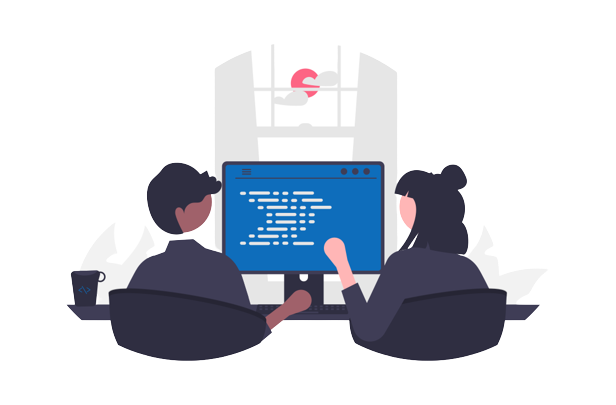
Javascript Console is one of the most used tools in debugging. Bring your Javascript Console knowledge to a next level, you’ll be much faster, and more productive. Explore 5 things you didn’t know about Javascript Console!
Introduction
For Javascript developers, the console is one of the first things we explore. But it’s usually something that we don’t study in depth. We use the usual log
method of the console or the error
method but I’ve prepared a list of 5 console methods that I wish I had known when I started writing Javascript. Without further ado, let’s jump right in.
Console table method
Logging primitive types with the log method is ok and pretty readable. But what about objects
or arrays
that have a lot of properties or elements? What if you want to see them in a table and easily compare them? However, if you are interested in learning more about array methods, check out my post.
You can use the following table method to get a nice preview of the object or array as a table in your browser console.
class Person {
constructor(firstName, lastName, job, hobby, city) {
this.firstName = firstName;
this.lastName = lastName;
this.job = job;
this.hobby = hobby;
this.city = city;
}
}
const person1 = new Person('Djordje', 'Arsenovic', 'Web developer', 'blog', 'Belgrade'),
person2 = new Person('John', 'Smith', 'Architect', 'fishing', 'Boston'),
person3 = new Person('Jane', 'Smith', 'Dentist', 'football', 'New York');
console.table([person1, person2, person3]);
It’s going to generate a preview like this.
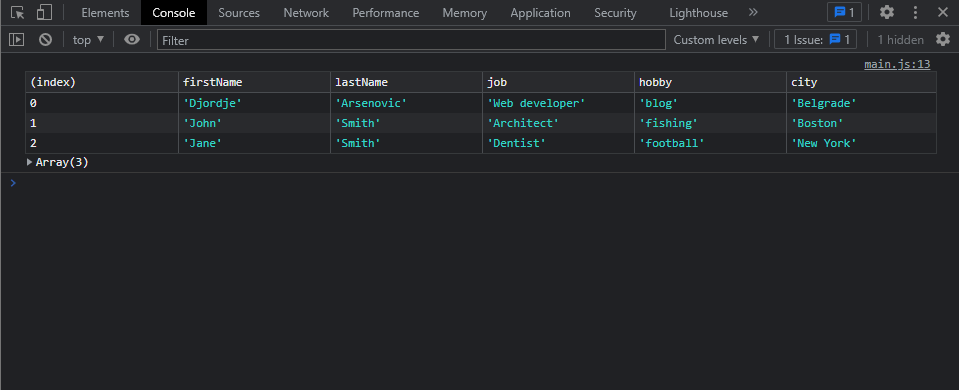
However, another perk is that you can show only the object properties you want. So if for example, you would like to show only first and last names you can do that by adding one more parameter to the console.table()
call.
console.table([person1, person2, person3],['firstName', 'lastName']);
This will show you only the first and last names of all three persons on the screenshot below.
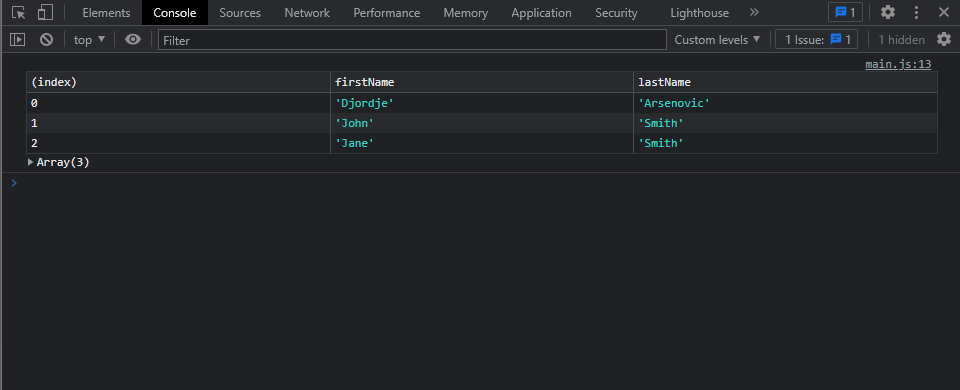
Console time method
This method is really useful for measuring the time for any process that you are running. MDN gave an example for showing an alert, while I wanted to give you a more real-world example.
const fetchPosts = async () => {
return await fetch('https://jsonplaceholder.typicode.com/posts')
.then(res => res.json());
}
(async () => {
console.time('Get the Posts');
const allPosts = await fetchPosts();
console.timeEnd('Get the Posts');
console.table(allPosts)
})()
In the code above I’ve used the async
functions to fetch the posts from JSONPlaceholder (which is a great tool by the way) and to show you the results I’ve used IIFE (Immediately Invoked Function Expression) because you can only await
inside async
function. So you can measure the time for the get request and when the data can be used.
Where you can use the console time method
You can use this method wherever you want but the best use cases I would use it:
- GET/POST requests
- For loop
- Some rendering that you are doing
Just to see where your application is spending the most time.
Console assert method
This method is interesting because, whenever you are in some doubt is something equal, you can test that by using the assert method. You can use this method wherever you want in javascript. The syntax is following
console.assert(2 === '2'); // false
console.assert(2 == '2'); // true
console.assert(typeof '2' === 'string'); // true
Where you can use the console assert method
When testing which numbers in the array are even? As you can see in the code below we have a bit different syntax we are passing one more argument, a message. The message will be displayed when the assertion fails.
const numbers = [2, 3, 4, 5, 6, 10, 240, 121];
numbers.forEach(number => {
console.log(`Testing is ${number} even?`);
console.assert(number % 2 === 0, `The number ${number} is not even!`);
});
This will yield the following console logs and errors.
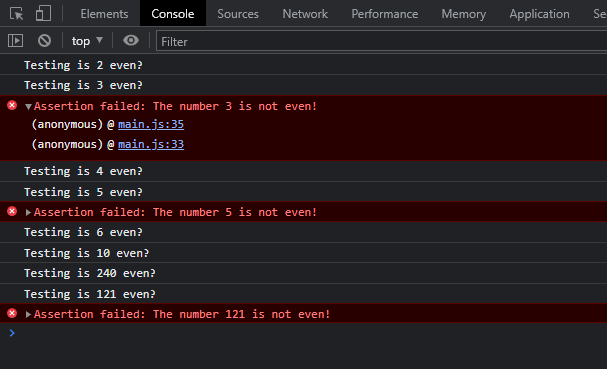
Console count method
Like the above console methods, this one too is useful for debugging our application or optimizing it. You can check how many times your function has been called. In a simple example like ours, it’s not very useful but there are cases when you have a lot of functions and classes, etc, and you really can’t follow up on what was called and how many times, especially if you are working with async functions.
const fetchPosts = async () => {
console.count('fetchPosts function');
return await fetch('https://jsonplaceholder.typicode.com/posts')
.then(res => res.json());
}
(async () => {
console.count('IIFE');
const allPosts = await fetchPosts();
setTimeout(async () => {
await fetchPosts();
console.count('From the timeout!')
}, 2000);
})();
So in the above code, we have our fetch posts async functions, and we have also set the timer to two seconds. We have also set up the count method to see which function fired how many times. That code above is going to give us output like this.
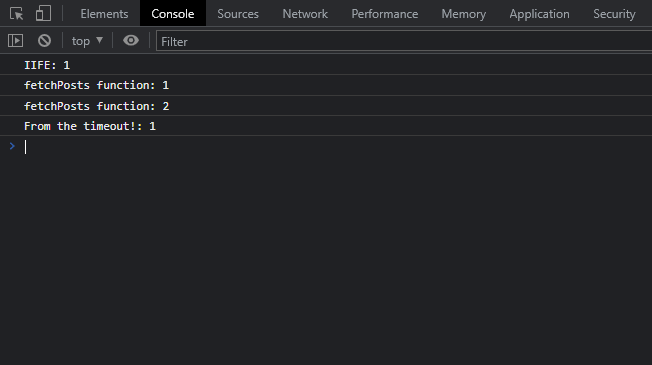
Console trace method
This method allows you to as its name says trace the code execution. You just need to place console.trace();
anywhere in your code but it’s most useful in functions, and see which function will be called and when. Like before we are going to use the same example with our async functions and the setTimeout.
const fetchPosts = async () => {
console.trace('fetchPosts function');
return await fetch('https://jsonplaceholder.typicode.com/posts')
.then(res => res.json());
}
(async () => {
const allPosts = await fetchPosts();
setTimeout(async () => {
await fetchPosts();
}, 2000);
})()
This code will produce this kind of trace
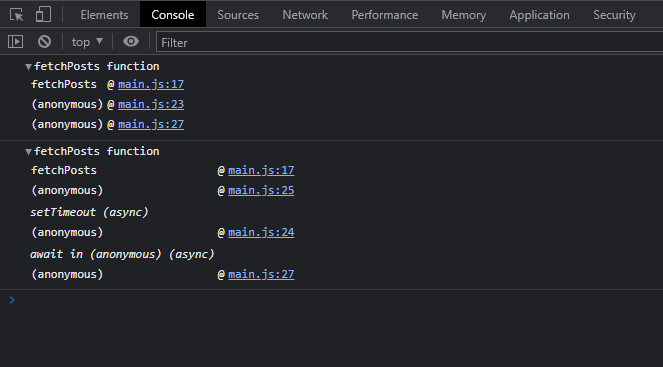
Where you can use the console trace method
As shown above, you can use this code if you have calls from one function to another function, and in the rabbit hole you go! 😀 I am just kidding, but my colleague used to call that kind of development “dream within a dream”. And so if you want to chase your dreams – functions, use the trace method from the console, thank me later!
Conclusion
Well, we are at the end of our journey with console, and it’s time for us to conclude this post. While the console is a pretty low-level debugging tool it can be pretty powerful when used right. So I dare you to try and use these methods in your day-to-day coding workflow, and try new ways how you can use them. If you do please let me down in the comments below your thoughts about this post and if you find some new ways to use the console that I forgot to mention.