Mastering JavaScript Array map & filter
Arrays are everywhere, in all programming languages. Master Javascript arrays using js map and js filter methods.
Introduction
In this post, we are going to explore Arrays in Javascript and the built-in methods that we can use to easily work with them. Arrays are everywhere in JS, whether you are working with Vanilla JS or with some framework like Vue or even libraries, you will face arrays sooner rather than later.
In JavaScript, arrays aren’t primitives but are instead Array
objects with the following core characteristics as per mdn documentation:
Now that we know the basic characteristics of JavaScript arrays, let’s kick off this topic with some built-in methods that we can use to manipulate JavaScript Arrays.
Using the JavaScript Map Method to Transform Your Arrays
One of the most used cases there is mapping through the array, right? Since there can be many elements inside Array. We need to loop through them in order to do some other operations. The most important difference between looping through an array with a map or with forEach is that map will create a new array.
Let’s take a look at the following example using an array map as an arrow function. The arrow function in the below example serves as the callback function that changes each element (x) by multiplying it by 2. Therefore new array has elements 2, 8, 18, and 32.
const array1 = [1, 4, 9, 16];
// Pass a function to map
const map1 = array1.map(x => x * 2);
console.table(map1);
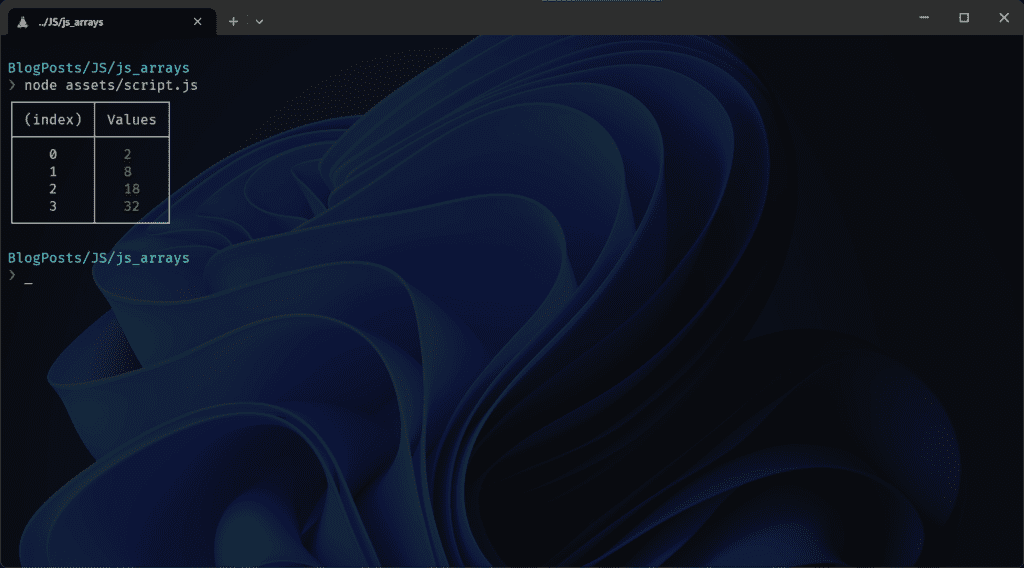
Let’s try one more example with the more complex elements in the array. We have an array of objects called employees, and we are trying to create a new array of all employees older than 40.
const employees = [
{
name: 'John Doe',
age: 41,
occupation: 'NYPD',
},
{
name: 'Jane Doe',
age: 26,
occupation: 'LAPD',
},
{
name: 'Robert Downey Jr.',
age: 48,
occupation: 'Iron Man',
},
];
const newEmployeesArr = employees.map((employee) => employee.age > 40);
console.table(newEmployeesArr);
If we use an Array map as above we are going to get the following result.
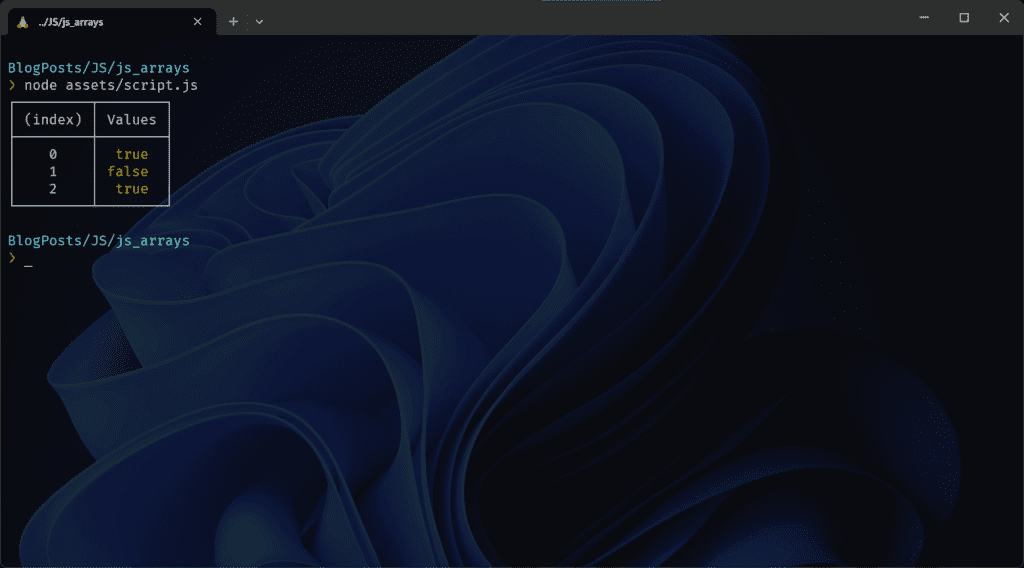
Warning❗
[true, false, true]
.If you expected to see an array of objects that have age property greater than forty, please keep reading! You will definitely like the next method!
When to use javascript map?
I use JS map when certain conditions are met, and those are:
So basically if you need to change all the elements of that array that you have and you don’t want to use the foreach
or for
loop you can do that with map
.
const employees = [
{
id: 1,
name: 'John Doe',
age: 41,
occupation: 'NYPD',
},
{
id: 2,
name: 'Jane Doe',
age: 26,
occupation: 'LAPD',
},
{
id: 3,
name: 'Robert Downey Jr.',
age: 48,
occupation: 'Iron Man',
},
];
const employeesMessageArr = employees.map((el) => {
el['message'] = `Hi my name is ${el.name} and I am ${el.occupation}`;
return el;
});
console.table(employeesMessageArr);
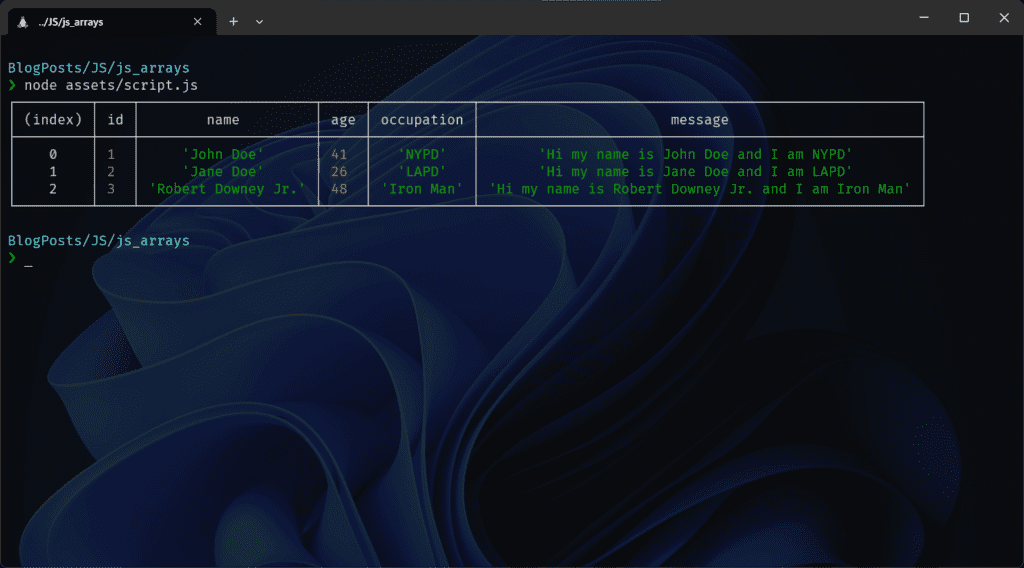
Like in the example above I wanted to create a new array where my employees have a message inside the object that I can use on their public profile page, something like a tagline, so in each object, I’ve injected a new property message. And using ES6 template strings I’ve created that message. Also, a good use-case can be when you are using react and you are looping through the array and rendering some JSX or even a component. Check out my tutorial for full-stack web applications with Laravel and React.
How to Use Arrow Functions to Filter Array of Objects in JavaScript?
As its name says it filters the array of elements by certain criteria. But in order to do that, firstly it needs to loop through the array, secondly, it needs to check the condition that you told him, lastly, it creates the new array with the elements that match your condition. Let’s look at the code below.
const employees = [
{
name: 'John Doe',
age: 41,
occupation: 'NYPD',
},
{
name: 'Jane Doe',
age: 26,
occupation: 'LAPD',
},
{
name: 'Robert Downey Jr.',
age: 48,
occupation: 'Iron Man',
},
];
const newEmployeesArr = employees.filter((employee) => employee.age > 40);
console.table(newEmployeesArr);
As you can see we are able to filter out all the employees that are older than forty. To do that we have used the array filter
the method along with the condition employee.age > 40
. Using the arrow function implicit return, it will automatically return all the employee objects where the age property is greater than forty.
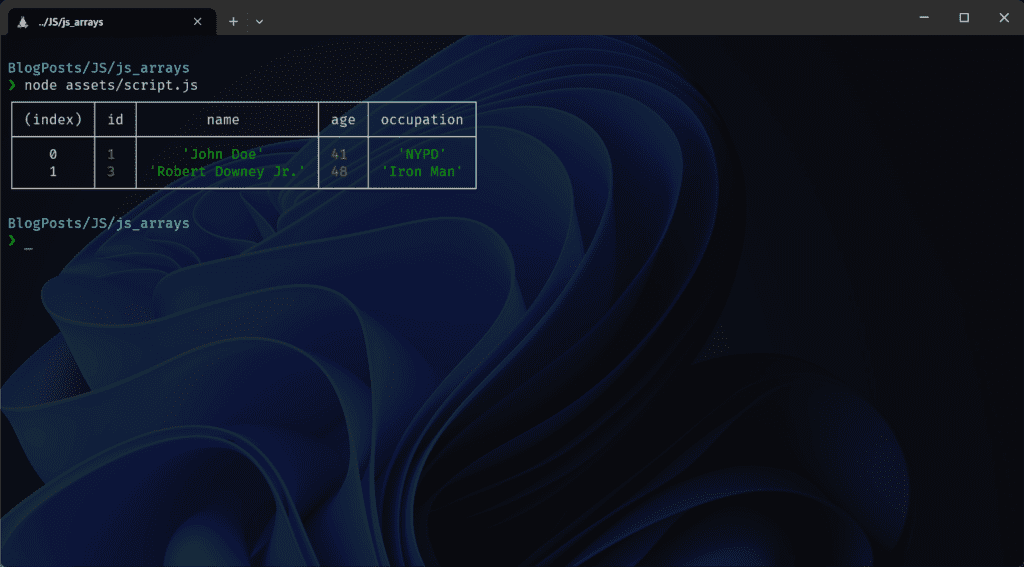
When to use JavaScript filter?
As you can see filter is really nifty method to use. It doesn’t replace your array, it creates a new one, which is very important for some use-cases. Let’s see when you can use the Javascript filter method.
How to use a filter method to search JavaScript array?
Use the JS filter to create a full-text search! Let’s say that you have an array of objects, and you want to search through that array, you can use the following code.
const employees = [
{
id: 1,
name: 'John Doe',
age: 41,
occupation: 'NYPD',
},
{
id: 2,
name: 'Jane Doe',
age: 26,
occupation: 'LAPD',
},
{
id: 3,
name: 'Robert Downey Jr.',
age: 48,
occupation: 'Iron Man',
},
];
const searchedArr = employees.filter((employee) => employee.name.includes('Doe'));
console.table(searchedArr);
In the example above we are using an object property name and the includes
a function to search through the employee array. String method includes will give true or false if the string includes in our case another string Doe
.
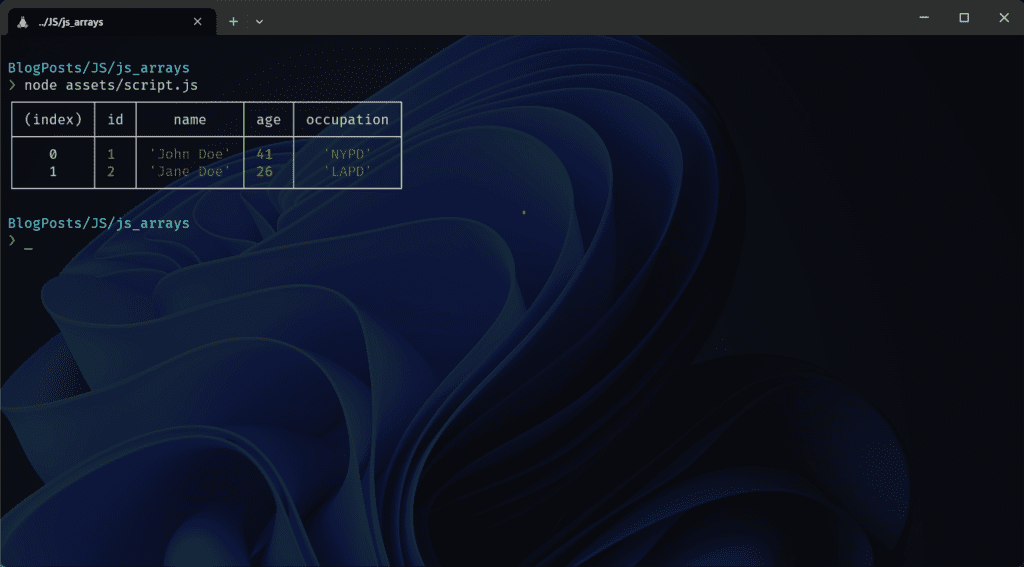
How to remove elements from JavaScript array?
Use the JS filter method when updating react state. React state is immutable, so creating a new array when filtering the previous state will be really handy. So let’s consider this example, you have the list of users like before, and you have just deleted an employee, you can filter her out by using the condition (employee) => employee.id !== 2
.
const employees = [
{
id: 1,
name: 'John Doe',
age: 41,
occupation: 'NYPD',
},
{
id: 2,
name: 'Jane Doe',
age: 26,
occupation: 'LAPD',
},
{
id: 3,
name: 'Robert Downey Jr.',
age: 48,
occupation: 'Iron Man',
},
];
const newEmployeesArr = employees.filter((employee) => employee.id !== 2);
Warning❗
!==2
, because I want to remove that user from the array. If I used === sign here it would give me only the user with an id of 2. And we want the opposite of that.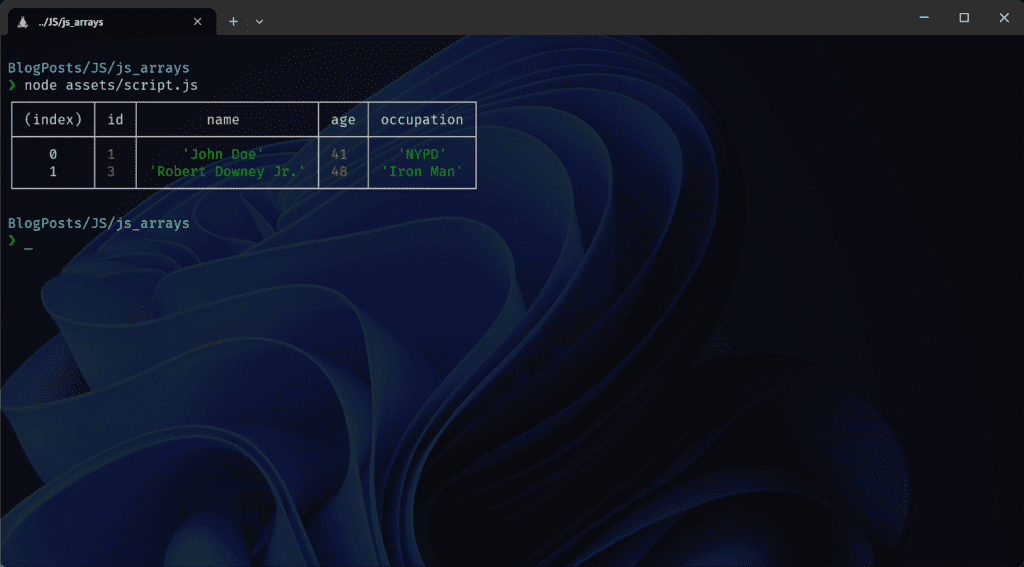
Conclusion
So both of these methods are good to have in your skill belt. They are used everywhere in JS both front-end and back-end. You should really get familiar with them because you are probably going to use them more than you think. Just remember, use the right tool for the right job.